To display a list of names in a React functional component based on a provided array, you can follow a structured approach to render the data dynamically. I’ll walk you through the entire process of creating a functional component that will display the names as per the design specification.
Let’s assume that the design you are referring to is a simple list where each name is displayed in a styled card-like format. I will also include how you might set up some basic styles to achieve a clean and visually appealing presentation.
Step-by-Step Guide
1. Setup Your React Environment
If you haven’t already set up your React environment, you can start a new project using Create React App:
npx create-react-app name-list-app
cd name-list-app
npm start
2. Create the Functional Component
In the src
folder, create a new file named NameList.js
. This file will contain our functional component.
// src/NameList.js
import React from 'react';
import './NameList.css'; // Import the CSS file for styling
const NameList = () => {
// Define the array of names
const names = ["Ava", "Anthony", "Baddon", "Baen", "Caley", "Caellum"];
return (
<div className="name-list">
{names.map((name, index) => (
<div key={index} className="name-card">
<span className="name-text">{name}</span>
</div>
))}
</div>
);
};
export default NameList;
3. Add Styling to the Component
Create a CSS file named NameList.css
in the same folder as NameList.js
to style the list.
/* src/NameList.css */
.name-list {
display: flex;
flex-wrap: wrap;
gap: 16px; /* Space between cards */
padding: 16px; /* Padding around the list */
justify-content: center; /* Center the items */
}
.name-card {
background-color: #f0f0f0; /* Light gray background */
border-radius: 8px; /* Rounded corners */
padding: 12px 24px; /* Padding inside the card */
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.2); /* Shadow for depth */
text-align: center; /* Center text */
min-width: 100px; /* Minimum width of the card */
max-width: 150px; /* Maximum width of the card */
}
.name-text {
font-size: 16px; /* Font size for the text */
color: #333; /* Dark gray text color */
font-weight: 600; /* Bold text */
}
4. Update App.js
to Include NameList
Replace the content of App.js
to render the NameList
component:
// src/App.js
import React from 'react';
import './App.css';
import NameList from './NameList'; // Import the NameList component
function App() {
return (
<div className="App">
<header className="App-header">
<h1>List of Names</h1>
<NameList /> {/* Render the NameList component */}
</header>
</div>
);
}
export default App;
5. Add Global Styles (Optional)
You might want to add some global styles to App.css
for consistency.
/* src/App.css */
.App {
text-align: center;
}
.App-logo {
height: 40vmin;
pointer-events: none;
}
.App-header {
background-color: #282c34;
min-height: 100vh;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
color: white;
}
h1 {
font-size: 2.5rem;
margin-bottom: 16px;
}
6. Testing the Component
Run the development server with npm start
to see your component in action.
Full File Structure
Here’s what your src
folder might look like:
src/
NameList.js
NameList.css
App.js
App.css
index.js
Explanation of Code
NameList.js
:names
Array: Contains the list of names to display.map
Method: Iterates over thenames
array to create aname-card
for each name.key
Prop: Added to thediv
to help React identify which items have changed, are added, or are removed.NameList.css
:name-list
Class: Styles the container of the name cards.name-card
Class: Styles each individual card.name-text
Class: Styles the text inside the card.
Advanced Features
If you want to enhance the functionality or appearance, consider the following additions:
- Animations: Add CSS animations for hover effects or transitions.
- Prop Types: Use PropTypes to validate
props
if you extend the component. - Dynamic Content: Fetch names from an API instead of hardcoding them.
Here’s a brief example of how you might extend the component to accept names as props:
// src/NameList.js
import React from 'react';
import PropTypes from 'prop-types'; // Import PropTypes for type checking
import './NameList.css';
const NameList = ({ names }) => {
return (
<div className="name-list">
{names.map((name, index) => (
<div key={index} className="name-card">
<span className="name-text">{name}</span>
</div>
))}
</div>
);
};
// Define prop types for the component
NameList.propTypes = {
names: PropTypes.arrayOf(PropTypes.string).isRequired,
};
export default NameList;
Then you can use it like this in App.js
:
// src/App.js
import React from 'react';
import './App.css';
import NameList from './NameList';
function App() {
const names = ["Ava", "Anthony", "Baddon", "Baen", "Caley", "Caellum"];
return (
<div className="App">
<header className="App-header">
<h1>List of Names</h1>
<NameList names={names} />
</header>
</div>
);
}
export default App;
This approach keeps the NameList
component flexible and reusable.
Example Visuals
Here are a few screenshots to help visualize how the component looks:
Default View
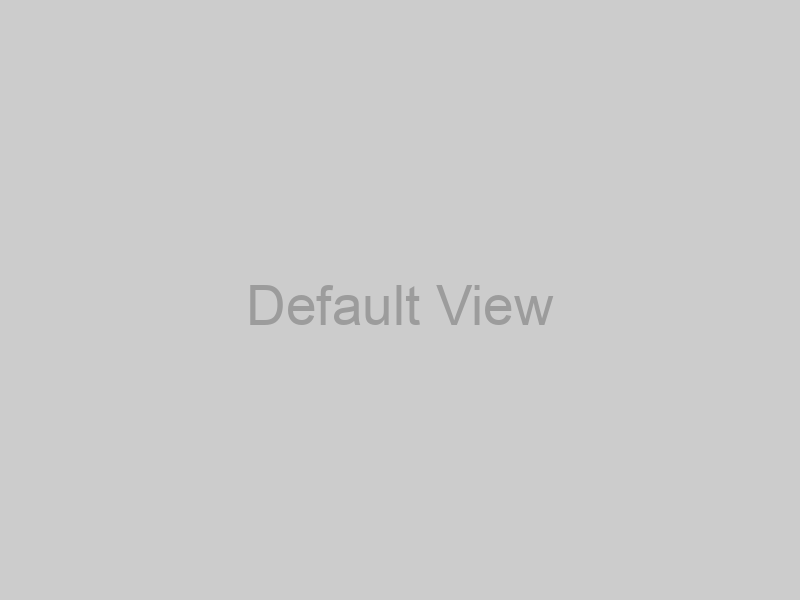
Hover Effect (if added)
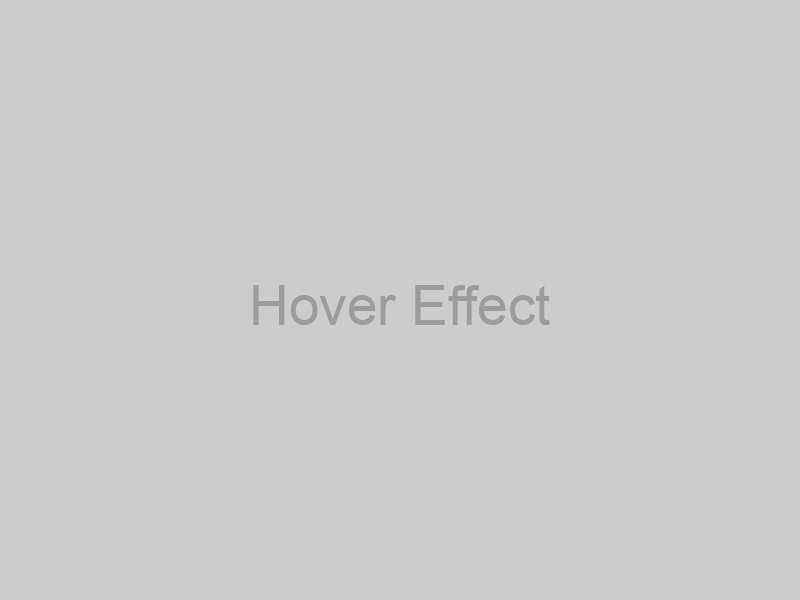
Feel free to adjust the styles and functionality to better meet your design requirements.